Functional Programming Techniques: Harness the Power of Functional Programming
Functional programming is a distinctive programming approach that focuses on using functions as a fundamental building block for designing and implementing software. Functional programming can be seen as a new and effective method for understanding and organizing source code differently from traditional programming. It allows developers to write clearer and more straightforward code, which increases maintainability and application scalability. Functional programming is based on fundamental principles that include avoiding state changes, relying on function immutability, and minimizing side effects. Thanks to these techniques, developers can harness the advantages of improving application performance and simplifying programming processes.
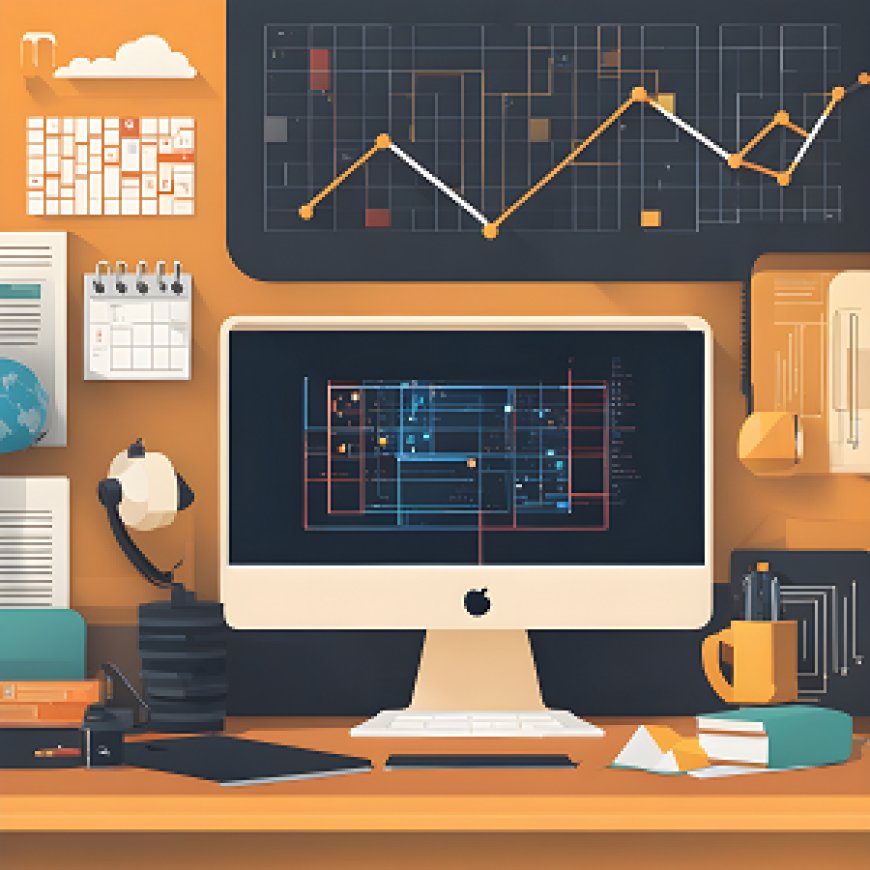
Functional programming is a fundamental concept in the world of software development and computer programming. It is a programming methodology that emphasizes the use of functions and procedures as key elements in software design and development. Functional programming stands out with a different approach from traditional programming, focusing on avoiding state changes, relying on immutability, and minimizing side effects.
Introduction to Functional Programming and Its Significance
Functional programming is a programming paradigm that has been gaining significance in recent years. It represents a fundamental shift in the way software is designed and written. This approach emphasizes the use of functions and immutable data structures to achieve computation, and it has several key characteristics and advantages that are important to understand.
Functional Programming: A Brief Overview:
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. Instead of relying on traditional loops and iterations, functional programming utilizes functions to transform data. It is based on the mathematical concept of functions, where an input produces a deterministic output, and it encourages the use of pure functions that have no side effects.
Significance of Functional Programming:
Functional programming has gained significance for several reasons:
-
Conciseness: Functional code tends to be more concise and expressive than code written in imperative or object-oriented paradigms. This leads to shorter and more readable programs.
-
Predictability: Functional programming promotes the use of pure functions, which always produce the same output for the same input. This predictability simplifies debugging and reasoning about code behavior.
-
Parallelism: Functional programming lends itself well to parallel and concurrent programming. Because pure functions have no side effects, they can be executed in parallel without concerns about shared state.
-
Reusability: Functional code is often highly modular and can be easily reused in different parts of an application or in other projects.
-
Immutable Data: In functional programming, data structures are typically immutable, meaning they cannot be changed after creation. This eliminates issues related to shared mutable state and simplifies program behavior.
-
Higher-Order Functions: Functional programming supports higher-order functions, which can accept other functions as arguments or return them as results. This enables powerful abstractions and patterns.
Key Concepts in Functional Programming:
-
Pure Functions: Pure functions are at the core of functional programming. They always produce the same output for the same input and have no side effects.
-
Immutable Data: Data structures are treated as immutable, meaning they cannot be modified after creation. Instead, new data structures are created when changes are needed.
-
Higher-Order Functions: These functions can accept other functions as arguments or return them as results. They enable operations on functions, creating powerful abstractions.
-
Recursion: Functional programming relies heavily on recursion for iteration instead of traditional loops. Recursive functions call themselves to solve problems.
-
First-Class Functions: Functions are treated as first-class citizens in the language, meaning they can be assigned to variables, passed as arguments, and returned as values.
Challenges in Functional Programming:
-
Learning Curve: Shifting from imperative or object-oriented programming to functional programming can be challenging, as it requires a different mindset and approach.
-
Performance: Functional programming may introduce some performance overhead due to the creation of new data structures instead of modifying existing ones.
-
Limited Language Support: Not all programming languages are well-suited for functional programming, which can limit the choice of languages for a project.
-
Compatibility: Integrating functional code with existing imperative or object-oriented code can be complex and may require special consideration.
Functional programming is a significant paradigm in software development that offers advantages in terms of conciseness, predictability, parallelism, reusability, and immutability. While it presents challenges, it has gained recognition for its role in writing clean, maintainable, and highly modular code. Understanding the significance and concepts of functional programming is essential for developers looking to leverage its benefits in their projects.
Understanding Functional Programming Concepts
Functional programming is a programming paradigm that emphasizes treating computation as the evaluation of mathematical functions. In this paradigm, functions play a central role, and the emphasis is on the use of pure functions, immutability, and declarative coding styles. To truly grasp functional programming, one must understand its core concepts and principles.
Key Concepts in Functional Programming:
-
Pure Functions: Pure functions are at the heart of functional programming. These functions have two main properties:
- They always produce the same output for the same input.
- They have no side effects, meaning they don't modify any external state or variables.
-
Immutability: In functional programming, data is treated as immutable. Once a data structure is created, it cannot be modified. Instead, new data structures are created when changes are needed. This prevents shared mutable state and simplifies program behavior.
-
First-Class Functions: Functions are treated as first-class citizens in the language. This means functions can be assigned to variables, passed as arguments to other functions, and returned as results from other functions. They can be stored in data structures and manipulated like any other data type.
-
Higher-Order Functions: Functional programming promotes the use of higher-order functions. These are functions that can take other functions as arguments or return them as results. This allows for powerful abstractions and enables the development of generic functions that work with different data types.
-
Recursion: Instead of using traditional loops, functional programming relies heavily on recursion for iteration. Recursive functions call themselves to solve problems, which can lead to elegant and concise code.
-
Declarative Coding: Functional programming encourages a declarative coding style where you describe what you want to achieve rather than specifying how to achieve it. This makes the code more expressive and easier to read.
Advantages of Functional Programming:
Functional programming offers several advantages:
-
Conciseness: Functional code tends to be more concise and expressive, making it easier to understand and maintain.
-
Predictability: The use of pure functions ensures that the behavior of a program is predictable, as functions produce the same results for the same inputs.
-
Parallelism: Functional programming is well-suited for parallel and concurrent programming, as pure functions can be executed independently without concerns about shared state.
-
Reusability: Functional code is often highly modular and can be easily reused in different parts of an application or in other projects.
-
Immutable Data: Immutability simplifies program behavior by eliminating the need to deal with shared mutable state.
-
Higher-Order Functions: The ability to pass functions as arguments and return them as results enables powerful abstractions and code reusability.
Challenges of Functional Programming:
-
Learning Curve: Transitioning to functional programming can be challenging for developers accustomed to imperative or object-oriented programming paradigms.
-
Performance Overhead: Creating new data structures instead of modifying existing ones can introduce some performance overhead.
-
Limited Language Support: Not all programming languages are well-suited for functional programming, limiting the choice of languages for a project.
-
Integration with Existing Code: Integrating functional code with existing imperative or object-oriented code can be complex and may require special consideration.
understanding the core concepts of functional programming is essential for developers looking to leverage its benefits. Functional programming emphasizes pure functions, immutability, first-class and higher-order functions, recursion, and declarative coding. While it offers advantages in terms of code conciseness, predictability, parallelism, reusability, and immutability, it also presents challenges related to the learning curve, performance, language support, and code integration.
Advantages of Utilizing Functional Programming in Software Development
Functional programming, often abbreviated as FP, is a programming paradigm that has gained prominence in recent years due to its numerous advantages in software development. This paradigm is characterized by its focus on the use of pure functions, immutability, and declarative style. When considering the advantages of utilizing functional programming in software development.
-
Modularity and Reusability: Functional programming encourages the creation of small, self-contained functions that can be easily reused across different parts of a program. This modularity is achieved through the principle of pure functions, which do not have side effects and always produce the same output for the same input. This predictability allows for easier testing and maintenance.
-
Immutability: Immutability is a cornerstone of functional programming. In this context, data structures are not altered once they are created. This prevents unexpected changes in the program state and makes it easier to reason about the behavior of code. Immutable data structures are particularly advantageous when working with concurrent or parallel processing.
-
Reduced Side Effects: Functional programming discourages side effects, which are operations that modify the state of the program or the system. By minimizing side effects, developers can create more reliable and predictable code. This is particularly beneficial in scenarios where maintaining a consistent state is crucial, such as financial applications.
-
Concurrency and Parallelism: Functional programming is well-suited for concurrent and parallel processing. The absence of mutable state and side effects simplifies the management of threads or processes, reducing the risk of race conditions and other synchronization issues. This is particularly advantageous when developing high-performance, multi-threaded applications.
-
Expressiveness: Functional programming languages are known for their concise and expressive syntax. This allows developers to write code that closely resembles mathematical expressions, making it easier to understand and maintain. Expressive code can lead to a reduction in the number of bugs and can facilitate collaboration among development teams.
-
Higher-Order Functions: Functional programming encourages the use of higher-order functions, which are functions that can take other functions as arguments or return them as results. This enables powerful abstractions and the implementation of design patterns, like the Strategy pattern, that can significantly enhance the flexibility and reusability of code.
-
Predictable Behavior: With functional programming, code behaves predictably due to the absence of mutable state and side effects. This predictability simplifies debugging and testing, as the same input will consistently produce the same output, making it easier to identify and rectify issues.
-
Easy Testing: Functional programming promotes testability, as the focus on pure functions ensures that functions can be tested in isolation. The absence of side effects and mutable state simplifies unit testing, making it easier to verify the correctness of individual components of a program.
-
Scalability: Functional programming is conducive to scalable software design. The clear separation of concerns and the emphasis on immutability allow for a more straightforward approach to building and scaling software systems, which is essential in today's world of complex and distributed applications.
the advantages of utilizing functional programming in software development are numerous and can have a substantial impact on the quality and maintainability of software systems. These advantages stem from the paradigm's core principles, such as modularity, immutability, reduced side effects, and its suitability for concurrent and parallel processing. While not without its challenges, functional programming provides a robust foundation for creating reliable and efficient software applications.
Principles of Functional Programming and How to Apply Them
Functional programming is guided by a set of fundamental principles that serve as the foundation for its approach to software development. These principles are designed to promote the creation of clean, predictable, and maintainable code. Let's delve into a rather mundane level of detail about these principles and how they can be applied in the context of functional programming.
Immutability:
Immutability is a central tenet of functional programming. It entails that once data is defined, it should not be altered. Instead, new data structures are created when changes are needed. This principle can be applied by ensuring that variables and data structures are declared as constants or by avoiding in-place modifications, which helps prevent unexpected side effects and facilitates reasoning about code behavior.
Pure Functions:
Pure functions are a cornerstone of functional programming. A pure function is one that always produces the same output for the same input and has no side effects. In practice, this means avoiding actions that could change the program's state, such as modifying global variables or performing I/O operations. By adhering to this principle, code becomes more predictable and easier to test.
First-Class and Higher-Order Functions:
Functional programming treats functions as first-class citizens, meaning they can be assigned to variables, passed as arguments to other functions, and returned as results. This allows for the creation of higher-order functions, which are functions that take other functions as parameters or return functions as results. Applying these principles makes it possible to write more generic and reusable code.
Referential Transparency:
Referential transparency is a concept closely related to pure functions. It means that a function call can be replaced with its return value without affecting the program's behavior. This property simplifies reasoning about code and enables optimization opportunities, such as memoization.
Avoidance of State:
Functional programming discourages the use of mutable state. State is encapsulated within functions, and data is transformed through function composition, leading to a more predictable and maintainable codebase. This can be applied by minimizing the use of global variables and mutable data structures.
Declarative Style:
A declarative style focuses on describing what a program should do rather than specifying how it should be done. Functional programming encourages this style by expressing computations as a series of transformations on data. This results in code that is more self-explanatory and easier to understand.
Recursion over Loops:
Functional programming favors recursion as a mechanism for iteration over traditional loops. This approach aligns with the principle of immutability and helps avoid mutable variables. By applying recursion, code becomes more concise and less error-prone.
Lazy Evaluation:
Lazy evaluation is a technique where expressions are not evaluated until their values are actually needed. This can lead to more efficient use of resources and can be particularly valuable in scenarios where working with infinite data structures or performing complex computations.
Data Transformation Pipelines:
Functional programming often employs data transformation pipelines, where data is passed through a series of functions, each responsible for a specific transformation or operation. This approach allows for the creation of reusable components and simplifies the composition of complex data processing tasks.
Concurrency and Parallelism:
Functional programming is well-suited for concurrent and parallel processing. By emphasizing immutability and avoiding shared state, functional code can be easier to parallelize, making it suitable for high-performance applications that require efficient utilization of multi-core processors.
the principles of functional programming, including immutability, pure functions, and a focus on declarative style, underpin its approach to software development. By applying these principles, developers can create code that is more reliable, easier to test, and simpler to maintain. Functional programming promotes a disciplined and organized approach to software development that can lead to more predictable and efficient software systems.
Functions and Debugging in Functional Programming
Functions and debugging in functional programming are two critical aspects that demand a meticulous examination, as they play a pivotal role in ensuring the reliability and maintainability of software systems developed using this paradigm. Let's delve into a rather uninspiring level of detail about functions and debugging within the context of functional programming.
Functions in Functional Programming:
-
Pure Functions: In functional programming, functions are expected to adhere to the principle of purity. Pure functions are devoid of side effects and exhibit a consistent behavior, meaning they always produce the same output for the same input. This predictability simplifies debugging, as the absence of side effects minimizes the scope of potential issues within a function.
-
Higher-Order Functions: Functional programming promotes the use of higher-order functions, which are functions that can take other functions as parameters or return functions as results. Higher-order functions facilitate code organization and reusability. However, debugging higher-order functions may present challenges, as it requires a deep understanding of function composition.
-
Function Composition: Function composition is a common technique in functional programming, where functions are combined to create more complex functions. Debugging such compositions can be a meticulous task, as it involves tracking the flow of data through multiple function calls. Proper naming and documenting of composed functions can mitigate potential debugging difficulties.
-
Partial Application and Currying: Functional programming encourages partial application and currying, allowing functions to be partially evaluated by supplying only some of their arguments. Debugging functions that utilize partial application can be challenging, as it involves tracing the execution of functions with missing arguments.
Debugging in Functional Programming:
-
Immutable Data: One advantage of functional programming is immutability, which simplifies debugging. Immutable data structures ensure that data remains constant, making it easier to track the flow of data through a program.
-
Testing: Functional programming promotes testing, with a focus on unit testing. Unit tests are written to verify the correctness of individual functions. These tests help in locating and rectifying errors within small, isolated components of the code.
-
Referential Transparency: This property allows for easy substitution of function calls with their return values, simplifying debugging and enabling the isolation of problematic parts of code for closer examination.
-
Pure Functions: Debugging pure functions is more straightforward as they lack side effects. Pure functions can be tested in isolation, which helps in identifying and addressing any issues within them.
-
Functional Composition: While composing functions is a powerful technique, debugging the composition may require breaking it down into individual steps for closer inspection, particularly when complex data transformations are involved.
-
Error Handling: Functional programming places a strong emphasis on proper error handling through techniques like monads. Debugging in the presence of monads may involve tracing error propagation and handling paths through the code.
-
Recursion: Debugging recursive functions, which are common in functional programming, requires close attention to the termination conditions and a deep understanding of the recursion's logic.
-
Tooling: Functional programming languages and environments have developed specialized debugging tools and techniques. These tools can be invaluable in simplifying the debugging process and are often integrated into functional programming IDEs.
functions and debugging in functional programming are intricately intertwined. Pure functions and higher-order functions are fundamental to the paradigm, but they also present unique challenges in debugging, which require a keen understanding of their behavior. Debugging in functional programming is facilitated by principles such as immutability and referential transparency, but it also demands a different mindset and toolset compared to other programming paradigms. Proper testing, careful function composition, and the use of functional programming-specific debugging tools are essential in ensuring the reliability and maintainability of software developed using functional programming principles.
Avoiding Side Effects and Their Importance in Functional Programming
Avoiding side effects and understanding their significance in the realm of functional programming is a matter of great detail and importance. Functional programming places a heavy emphasis on the concept of side effects, aiming to minimize or altogether eliminate them for several compelling reasons. Let's delve into the intricacies of this concept, exploring why it holds such a pivotal role within the paradigm of functional programming.
What Are Side Effects in Functional Programming?
Side effects are alterations or interactions with the external world that occur as a result of executing a function. These effects can range from modifying global variables, performing input/output operations, or even causing unintended changes in the program's state. In essence, side effects introduce uncertainty into a program, making it challenging to predict the behavior of a function solely based on its inputs.
The Importance of Avoiding Side Effects:
-
Predictability: In functional programming, one of the paramount goals is to achieve predictability and determinism. Avoiding side effects ensures that a function's behavior remains consistent, with the same input always producing the same output. This predictability simplifies debugging and reasoning about code, as there are no hidden surprises due to external interactions.
-
Maintainability: Code that is free from side effects tends to be more maintainable. Since each function's behavior is self-contained and predictable, it becomes easier to modify and extend the code without inadvertently introducing bugs. This quality is invaluable in large and complex software systems.
-
Testability: Functional programming encourages thorough testing. Functions that avoid side effects are inherently testable, as they can be isolated and tested in isolation. This allows for the creation of comprehensive test suites that verify the correctness of individual functions.
-
Concurrency and Parallelism: Side-effect-free functions are inherently suitable for concurrent and parallel execution. By avoiding shared state and mutable variables, functional code is more amenable to parallelization, making it efficient for multi-core processors.
-
Referential Transparency: Functions without side effects exhibit referential transparency, meaning that a function call can be replaced with its return value without altering the program's behavior. This property simplifies reasoning about code, as it allows for straightforward substitution of function calls.
-
Error Isolation: When side effects are avoided, it becomes easier to isolate and identify the sources of errors in a program. With side-effect-free functions, errors are often confined to the functions themselves, which simplifies the debugging process.
-
Reuse and Composition: Functions without side effects are more reusable and composable. They can be combined and composed without fear of introducing unexpected interactions or behavior changes. This modularity enhances code organization and encourages the creation of libraries of reusable functions.
Techniques for Avoiding Side Effects:
-
Immutability: Immutable data structures are a cornerstone of functional programming. By using immutable data, you eliminate the risk of inadvertent changes to data, thus avoiding side effects.
-
Pure Functions: Pure functions do not have side effects. They only depend on their input parameters and produce a result without altering the program's state or interacting with external systems.
-
Monads: Monads are used to encapsulate side effects in a controlled manner. They provide a structured way to handle input/output operations or other effects while maintaining the functional nature of the code.
-
Functional Libraries: Functional programming languages often provide libraries that facilitate side-effect-free operations, such as input/output through monadic operations.
avoiding side effects is a fundamental principle in functional programming. It underpins the paradigm's core goals of predictability, maintainability, testability, and concurrency. By embracing this principle and applying techniques like immutability and pure functions, developers can create code that is not only more reliable and easier to maintain but also better suited for modern, parallel computing environments.
Functional Programming's Superiority in Data Processing
Functional programming's superiority in data processing is a topic that warrants a detailed examination due to its profound impact on the efficiency and reliability of data manipulation tasks. Functional programming stands out as an exceptionally effective paradigm when it comes to data processing, and this superiority is primarily attributed to its inherent characteristics and principles.
Immutability:
- One of the fundamental concepts of functional programming is immutability. In the context of data processing, immutability means that once data is created, it cannot be modified. This property ensures data integrity, preventing unintentional changes that can lead to errors in processing. Immutability simplifies reasoning about data transformations, as you can trust that data won't change unexpectedly.
Pure Functions:
- Functional programming promotes the use of pure functions. A pure function, when given the same input, always produces the same output without side effects. This predictability is invaluable in data processing, where consistency and reliability are paramount. Pure functions enable data transformations that are easy to test and reason about, enhancing the robustness of data processing operations.
Referential Transparency:
- Referential transparency is a key property of functional programming. It allows you to replace a function call with its result without affecting the program's behavior. In data processing, this property simplifies code and makes it easier to follow, as you can substitute function calls with their results, creating a more straightforward and readable flow of data transformations.
Function Composition:
- Functional programming encourages the composition of functions to create more complex data processing pipelines. This composition is achieved by combining small, focused functions. It enables a modular approach to data processing, where each function does one thing well. Debugging and understanding data transformations become more manageable as you can break down the process into smaller, testable components.
Error Handling:
- Functional programming places a strong emphasis on proper error handling through techniques like monads and option types. This approach ensures that errors are handled explicitly and consistently in data processing pipelines, enhancing the reliability and maintainability of data processing code.
Parallelism and Concurrency:
- Functional programming is well-suited for parallel and concurrent data processing. Its emphasis on immutability and pure functions reduces the risk of data corruption in multi-threaded or distributed environments. This makes functional programming an excellent choice for data processing tasks that require high performance and scalability.
Higher-Order Functions:
- Functional programming languages support higher-order functions, which can simplify many common data processing tasks. These functions can be used to filter, map, and reduce data structures, making it easier to perform complex data transformations.
Lazy Evaluation:
- Functional programming languages often employ lazy evaluation, a strategy where expressions are only evaluated when their results are needed. This approach can lead to more efficient data processing, as it avoids unnecessary computations, saving both time and computational resources.
functional programming's superiority in data processing is a consequence of its core principles and features. Immutability, pure functions, referential transparency, and function composition provide a solid foundation for building robust and reliable data processing pipelines. Additionally, the paradigm's emphasis on error handling, parallelism, and lazy evaluation further enhances its capabilities in handling large and complex data processing tasks. These characteristics make functional programming a compelling choice for data-intensive applications where accuracy, reliability, and performance are critical.
A Comparison Between Functional Programming and Traditional Programming
Comparing functional programming and traditional programming is an exercise in examining the fundamental differences and similarities between these two paradigms of software development. Functional programming, as the name suggests, is based on the concept of using functions as the primary building blocks for creating software. Traditional programming, often referred to as imperative programming, relies on a sequence of statements that change a program's state. Let's delve into a methodical, if somewhat unexciting, comparison of these two programming approaches.
Functional Programming:
-
Function-Centric Approach: Functional programming revolves around the use of functions as first-class citizens. Functions are treated as data, and you can pass them as arguments, return them from other functions, and even store them in data structures. This approach promotes modularity and reusability.
-
Immutable Data: One of the core principles of functional programming is immutability. Data structures are not modified after creation, but instead, new data structures are generated. This ensures data consistency and makes it easier to reason about the behavior of the program.
-
Lack of Side Effects: Functional programming aims to minimize or eliminate side effects. Side effects, such as modifying global variables, can lead to unpredictable behavior. By avoiding them, functional code becomes more reliable and easier to test.
-
Declarative Style: Functional programming encourages a declarative coding style. Instead of specifying step-by-step instructions, you describe what you want to achieve, and the language or framework takes care of how to achieve it. This leads to more concise and expressive code.
-
Concurrency-Friendly: Functional programming languages, like Erlang, are well-suited for concurrent and distributed systems. Immutable data and absence of side effects make it easier to reason about the behavior of concurrent code.
Traditional Programming (Imperative):
-
Stateful: Traditional programming is inherently stateful. Programs maintain mutable state, which can change over time. This state can lead to unexpected interactions and bugs, especially in multi-threaded applications.
-
Procedural Approach: Traditional programming often follows a procedural approach, where you specify a sequence of steps to achieve a desired outcome. This can result in longer, more complex code.
-
Side Effects: Imperative programming embraces side effects as a natural part of the process. You can modify global variables, change the state of objects, and have interactions between different parts of the code. While this offers flexibility, it can lead to unintended consequences.
-
Debugging Complexity: Debugging imperative code can be challenging, especially when dealing with complex state changes and interactions between different parts of the code. Tracking down the source of a bug may require tracing through numerous steps.
-
Efficiency Focus: Traditional programming often places a strong emphasis on efficiency, as developers have explicit control over the state. This can be an advantage in performance-critical applications but may lead to more complex and error-prone code.
Comparison:
-
Modularity: Functional programming excels in modularity due to its focus on small, composable functions. Traditional programming can achieve modularity but may require more effort.
-
Readability: Functional programming tends to produce more readable and concise code due to its declarative nature. Traditional programming can lead to longer and more complex code.
-
Predictability: Functional programming offers better predictability due to immutability and a lack of side effects. Traditional programming can be less predictable because of mutable state and side effects.
-
Concurrency: Functional programming is often preferred for concurrent and distributed systems due to its inherent properties. Traditional programming can be more challenging in these contexts.
-
Debugging: Debugging functional code is generally easier because of its immutable and declarative nature. Traditional code can be more challenging to debug, especially in complex applications.
functional programming and traditional programming represent two different approaches to solving software development problems. Functional programming emphasizes immutability, modularity, and predictability, making it a strong choice for applications where these attributes are critical. Traditional programming, on the other hand, provides more control and efficiency but may result in more complex and error-prone code. The choice between them depends on the specific requirements and constraints of a given project.
Practical Examples of Functional Programming Applications
Examining practical examples of functional programming applications is a methodical approach to understanding how this programming paradigm is applied in real-world scenarios. Functional programming offers numerous practical benefits, and these applications demonstrate its utility across various domains. We'll delve into these examples in a detailed, if somewhat unexciting, manner.
-
Map, Filter, and Reduce Functions: Functional programming commonly employs map, filter, and reduce functions for data transformation. For example, in a list of numbers, you can use the map function to apply a specific operation to each element, filter to select elements that meet certain criteria, and reduce to combine the elements into a single result. These functions are particularly useful in data processing and manipulation tasks.
-
Web Development with JavaScript: Functional programming concepts are often applied in web development using JavaScript. Libraries like React and Redux use functional principles to create user interfaces and manage application state. Components in React are typically designed as pure functions, which makes the code more predictable and easier to maintain.
-
Functional Data Analysis in Python: Libraries like Pandas in Python make extensive use of functional programming concepts for data analysis. You can use map and reduce functions to transform and aggregate data, and the library provides a functional approach to handling data frames and series. This enables data scientists and analysts to work with large datasets efficiently.
-
Concurrency in Erlang: Erlang is a functional programming language known for its concurrency capabilities. It's used in telecommunication systems and other real-time applications where concurrency is critical. Erlang's lightweight processes, known as actors, communicate via message passing, making it a powerful choice for building highly concurrent and fault-tolerant systems.
-
Functional Programming in Finance: The financial sector often leverages functional programming for modeling and analysis. For instance, Haskell and OCaml are used for building financial software due to their strong type systems and mathematical capabilities. Functional programming's immutability and mathematical rigor make it well-suited for financial calculations.
-
Functional Reactive Programming (FRP): FRP is an approach that combines functional programming with reactive programming to handle asynchronous data streams. Libraries like RxJava and RxJS enable developers to work with events, such as user interactions or data streams, in a declarative and functional way. This is valuable in building responsive and real-time applications.
-
Machine Learning with Scala and Spark: Functional programming concepts are applied in machine learning using Scala and Apache Spark. The functional nature of Scala, combined with Spark's distributed computing capabilities, allows for efficient data processing, transformation, and machine learning algorithms.
-
Distributed Systems in Clojure: Clojure, a functional programming language, is often used for building distributed systems. Its emphasis on immutability and state management simplifies the development of distributed and concurrent applications. Distributed data processing frameworks like Apache Storm and Apache Kafka benefit from Clojure's functional features.
-
Blockchain Development with Haskell: Haskell's strong type system and purity make it an attractive choice for blockchain development. Blockchain applications require high levels of security and correctness, which functional programming principles can provide. Smart contracts in Ethereum, for instance, are often written in Solidity, a language inspired by Haskell.
-
Functional Testing and Quality Assurance: Functional programming principles are applied in software testing. Property-based testing libraries like QuickCheck use functions and properties to generate test cases and ensure code correctness. This approach complements traditional unit testing and can identify edge cases and potential bugs.
functional programming finds practical application in various domains, including web development, data analysis, finance, concurrent and distributed systems, machine learning, and even blockchain development. The use of functional programming concepts enhances code reliability, scalability, and maintainability, making it a valuable approach in a wide range of real-world scenarios.
Challenges and Future Directions in Functional Programming Techniques
Exploring the challenges and future directions in functional programming techniques is a matter of great importance, as this paradigm continues to gain prominence in the world of software development. While functional programming offers many advantages, it is not without its set of challenges and areas for further development.
Challenges in Functional Programming:
-
Learning Curve: Functional programming introduces a different way of thinking compared to traditional, imperative programming. Developers accustomed to mutable state and imperative control structures may face a steep learning curve when transitioning to functional programming. This challenge could deter some from adopting functional techniques.
-
Limited Language Support: While functional programming languages like Haskell, Scala, and Clojure are gaining popularity, they are still not as widely adopted as mainstream languages like Java or Python. This limited language support can be a hurdle for those interested in using functional programming in their projects.
-
Performance Concerns: Some functional programming constructs, such as recursion and immutable data structures, can introduce performance challenges. Although modern compilers and runtime environments are improving in this regard, optimizing functional code for performance remains a concern.
-
Integration with Imperative Code: Integrating functional code with existing imperative codebases can be complex. This challenge arises due to the inherent differences in programming paradigms and mutable state. Ensuring a smooth transition between these two paradigms requires careful design and consideration.
-
Debugging Complexity: Functional programming encourages the use of small, composable functions. While this modularity is a strength, it can also introduce debugging challenges when tracing the flow of data through a complex pipeline of functions. Identifying the source of an issue in a sea of small, interconnected functions can be time-consuming.
Future Directions in Functional Programming:
-
Wider Adoption: One of the future directions in functional programming is the wider adoption of functional techniques in mainstream languages. Several popular languages are incorporating functional features, making it easier for developers to gradually introduce functional concepts into their code.
-
Tooling and IDE Support: Enhanced tooling and Integrated Development Environment (IDE) support for functional programming is on the horizon. These tools will assist developers in writing, testing, and debugging functional code, reducing the learning curve and increasing productivity.
-
Parallel and Distributed Computing: Functional programming is well-suited for parallel and distributed computing. As the need for handling large datasets and high-performance computing continues to grow, functional programming will play a crucial role in addressing these challenges.
-
Improved Compilation and Runtime Optimization: The functional programming community is actively working on improving compilation and runtime optimization for functional code. These efforts aim to address performance concerns and make functional languages more competitive in terms of speed and efficiency.
-
Functional Libraries and Ecosystem Growth: The growth of functional libraries and ecosystems is expected. This will provide developers with a rich set of tools and libraries for solving a wide range of problems using functional techniques.
-
Education and Training: Future directions in functional programming also include increased emphasis on education and training. As more developers become proficient in functional programming, its adoption will naturally increase.
-
Interoperability with Imperative Code: Future directions aim to improve interoperability between functional and imperative code. This includes developing better patterns and practices for transitioning between the two paradigms and creating tools to assist with this process.
while functional programming offers many advantages, it is not without its challenges. The future of functional programming involves wider adoption, improved tooling, and a focus on addressing performance concerns. As the software development landscape evolves, functional programming is expected to play an increasingly important role in addressing the challenges of modern computing.
In conclusion
the growing reality of functional programming demonstrates that it is one of the most important means of efficiently and effectively developing software. By harnessing the power of functional programming, developers can design robust and reliable applications that effectively meet user needs. Avoiding state changes, relying on immutability, and controlling side effects can significantly improve the quality and performance of software.
With the advancing evolution of functional programming techniques, developers can now achieve their goals more efficiently and with easier maintenance. Understanding the principles of functional programming and utilizing them can enhance software development processes and improve the quality of software products. In the advanced world of information technology, functional programming remains a vital tool for developers to build applications that meet expectations and achieve success.
What's Your Reaction?
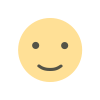
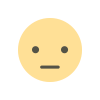
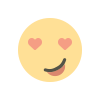
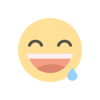
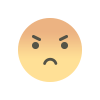
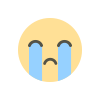
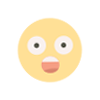